At some point while each of us were growing up, we wished that the adults in our lives would just disappear. They made our lives miserable with their arbitrary rules and restrictions, but they got to do all kinds of fun things. After all, how hard could it be to drive a car, and why did we need them to watch an R-rated movie or to cross the street?
Well, front end developers have a similar fantasy. Their wish is that one day, all back end developers will move out of their way and let them take control. Front end developers are responsible for the things people see and use. All the back end developers need to do is create REST APIs and HTTP endpoints that work and return well-formed JSON, and the front end developers do the rest.
One way you can get what you want in a timely manner is to go over to the dark side and become a full-stack developer, but where’s the fun in that? Well, there have been some interesting things happening in the world of cloud services, and soon, the tables may finally turn and put front end developers in the driver’s seat.
A new development that is benefiting front end developers is called serverless computing. Of course, the term “serverless computing” is deceptive. In this article, we take a look at the server behind the curtain, discuss the rise of serverless computing and what that means for front end development, and show you how you can get started.
What is Serverless Computing?
Serverless computing could just be a fad, and it might eventually join the long list of tech marketing buzzwords like Agile, virtualization, containers, DevOps, AI, machine learning, etc. In his article about serverless architecture, Mike Roberts defines serverless computing as applying to two different, but overlapping, models. These models are Backend as a Service (BaaS) and Functions as a Service (FaaS).
BaaS refers to third-party cloud services such as authentication, file storage, messaging, and data management. These services are accessible via an easy-to-use, web-based graphical user interface (GUI). Sometimes, they also have a command line interface (CLI) for building the services. The service also takes care of operational tasks such as maintenance, security, and scaling. Developers call these services directly from their client code via an HTTP endpoint and a rest API.
FaaS enables the developer to create a function that combines a number of exposed BaaS endpoints. These functions provide a container that can be deployed to provide specific backend services. The functions are triggered by an event such as a request sent from a client application.
In order to run the functions, FaaS services provide a compute platform and a gateway. The compute platform lets you write and deploy code that receives requests and performs the relevant tasks, such as user authentication, running queries against a database, and uploading files to storage. The gateway publishes the functions and provides an API proxy that receives requests from client applications, authenticates them, passes them to the compute platform for processing, and sends the response back to the client. In addition, all of the services provide a developer console, management tools, and various ways to analyze usage and performance.
Getting Started with Serverless Computing
One of the best places to start exploring serverless computing is Google’s Firebase service.
Below, we provide examples of how to use Firebase for BaaS and FaaS. Please note that the examples are all written in JavaScript and ReactJS.
Creating a Project
Before exploring the BaaS and FaaS aspects of Firebase, you must create a project. Creating a project with Firebase is very straightforward. First, you open the Firebase console web page and follow the instructions. Once you create the project, Firebase generates a JSON object that contains all of the configuration information. Then, you copy the object and include it as part of the project. Here is a sample of the generated code:
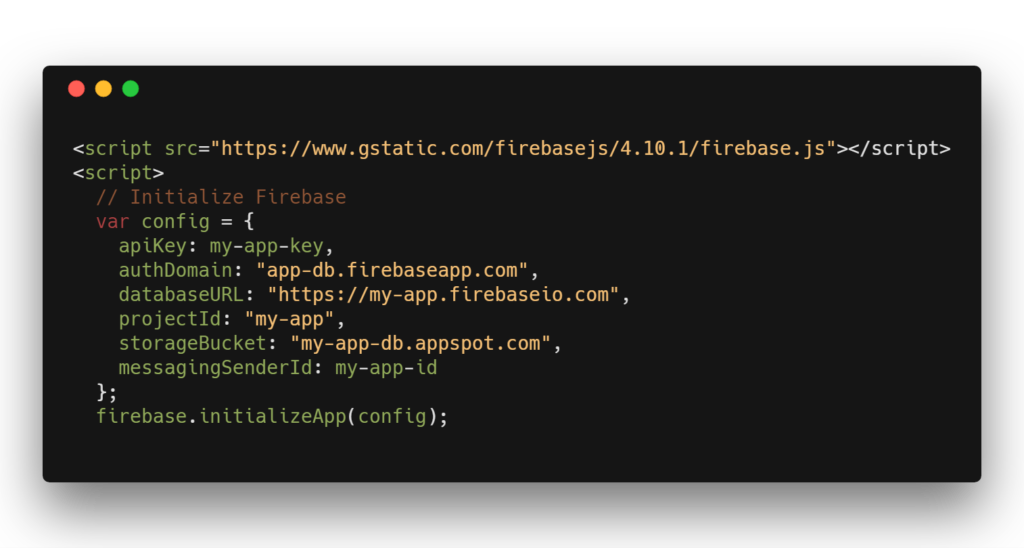
BaaS Services
Firebase provides a range of BaaS services, but for the purposes of this post, we will just review the authentication and data management services.
Authentication
Even if you do not use any other service, there is no excuse for not using commercial authentication. The provider has done most of the leg work, so you do not need to reinvent the wheel.
Firebase gives you everything you need to create and manage users. More importantly, it lets you create the rules that control permissions and data access. On the client side, it provides the Firebase UI library, which abstracts away the annoyance of building and maintaining client-side authentication code. For React developers, there is a Firebase UI React library that provides additional wrappers. The libraries allow you to create an object that lists the relevant authentication methods, and they show you how to handle the authentication process.
const uiConfig = {
signInOptions: [ firebase.auth.GoogleAuthProvider.PROVIDER_ID,
firebase.auth.FacebookAuthProvider.PROVIDER_ID ]
};
All you need to do is embed the Firebase authentication component in your class object’s render method.
class Login extends React.Component {
render() {
return
}
}
Data Management
Firebase provides a cloud-based document database called Firestore. A document is a JSON object that stores data in key-value pairs. Like other popular document databases such as MongoDB and CouchBase, Firestore lets you create and manage collections (groups of related documents like customer and sales data). In the following example, a user creates a product that manages a collection of users, and locates a document with the information for a specific member.
firebase.firestore().collection("members")
.where("member", "==", "jonnygold").get().then((snapshot) => {})
FaaS Services: Cloud Functions
Cloud Functions allow developers to write code that responds to specific events. These events can be handled by any Firebase or GCP service. For example, they can be used to handle authentication and query data, send messages related to changes for specific data, manipulate files, or integrate with third-party services. In the following example, the function is triggered when a user updates their profile:
exports.updateUser = functions.firestore
.document('users/{userId}')
.onUpdate(event => {
// Retrieves user document
let newVal = event.data.data();
// access relevant field
let name = newValue.name;
});
The Serverless Framework
In addition to exploring serverless offerings from Firebase, Google Cloud Platform, Microsoft Azure, and Amazon Web Services, you should also check out the Serverless Framework. It was built to provide tools for deploying and operating serverless architectures. The framework is vendor agnostic. It supports all the major vendors, but is designed so that you won’t get locked into a single provider’s solution. In fact, you can start building your app on any of the supported platforms, such as AWS, and at a later stage migrate it to a different platform, such as GCP or Azure. Serverless provides a command line interface for creating and deploying applications, and lets you use the language your project uses to handle configuration and application management.
Conclusion: Onwards and Upwards
We only scratch the surface of the serverless computing revolution in this short post. There is currently a lot of marketing hype about serverless computing, but it is true that this development represents a significant change in how we build apps. It gives front end developers the power and opportunity to build what they want, when they want, and how they want.
Firebase is a good place to start, but all of the platforms more or less offer the same services, and they all offer a developer/free tier to get you started. So whatever your idea is, you now have the tools to make it a reality.
In addition, once you get started you may want to migrate your serverless application to a new platform. This is where the Serverless Framework comes in. It will help you build a serverless application that does not depend on a single vendor’s tools or infrastructure. It will let you deploy your serverless system without the vendor lock-in that typifies cloud vendors and platforms.
To learn more about Applitools’ visual UI testing and application visual management (AVM) solutions, check out the tutorials on the Applitools website. To get started with Applitools, request a demo, or sign up for a free Applitools account.