Right before the end of 2022, Cypress surprised us with their new major release: version 12. There wasn’t too much talk around it, but in terms of developer experience (DX), it’s arguably one of their best releases of the year. It removes some of the biggest friction points, adds new features, and provides better stability for your tests. Let’s break down the most significant ones and talk about why they matter.
No more “detached from DOM” errors
If you are a daily Cypress user, chances are you have seen an error that said something like, “the element was detached from DOM”. This is often caused by the fact that the element you tried to select was re-rendered, disappeared, or detached some other way. With modern web applications, this is something that happens quite often. Cypress could deal with this reasonably well, but the API was not intuitive enough. In fact, I listed this as one of the most common mistakes in my talk earlier this year.
Let’s consider the example from my talk. In a test, we want to do the following:
- Open the search box.
- Type “abc” into the search box.
- Verify that the first result is an item with the text “abc”.
As we type into the search box, an HTTP request is sent with every keystroke. Every response from that HTTP request then triggers re-rendering of the results.
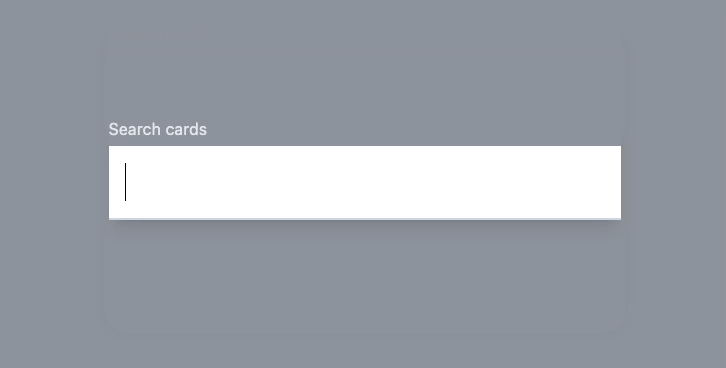
The test will look like this:
it('Searching for item with the text "abc"', () => {
cy.visit('/')
cy.realPress(['Meta', 'k'])
cy.get('[data-cy=search-input]')
.type('abc')
cy.get('[data-cy=result-item]')
.first()
.should('contain.text', 'abc')
})
The main problem here is that we ignore the HTTP requests that re-render our results. Depending on the moment when we call cy.get() and cy.first() commands, we get different results. As the server responds with search results (different with each keystroke), our DOM is getting re-rendered, making our “abc” item shift from second position to first. This means that our cy.should() command might make an assertion on a different element than we expect.
Typically, we rely on Cypress’ built-in retry-ability to do the trick. The only problem is that the cy.should() command will retry itself and the previous command, but it will not climb up the command chain to the cy.get() command.
It is fairly easy to solve this problem in versions v11 and before, but the newest Cypress update has brought much more clarity to the whole flow. Instead of the cy.should() command retrying only itself and the previous command, it will retry the whole chain, including our cy.get() command from the example.
In order to keep retry-ability sensible, Cypress team has split commands into three categories:
- assertions
- actions
- queries
These categories are reflected in Cypress documentation. The fundamental principle brought by version 12 is that a chain of queries is retried as a whole, instead of just the last and penultimate command. This is best demonstrated by an example comparing versions:
// Cypress v11:
cy.get('[data-cy=result-item]') // ❌ not retried
.first() // retried
.should('contain.text', 'abc') // retried
// Cypress v12:
cy.get('[data-cy=result-item]') // ✅ retried
.first() // retried
.should('contain.text', 'abc') // retried
cy.get() and cy.first() are commands that both fall into queries category, which means that they are going to get retried when cy.should() does not pass immediately. As always, Cypress is going to keep on retrying until the assertion passes or until a time limit runs up.
cy.session() and cy.origin() are out of beta
One of the biggest criticisms of Cypress.io has been the limited ability to visit multiple domains during a test. This is a huge blocker for many test automation engineers, especially if you need to use a third-party domain to authenticate into your application.
Cypress has advised to use programmatic login and to generally avoid trying to test applications you are not in control of. While these are good advice, it is much harder to execute them in real life, especially when you are in a hurry to get a good testing coverage. It is much easier (and more intuitive) to navigate your app like a real user and automate a flow similar to their behavior.
This is why it seems so odd that it took so long for Cypress to implement the ability to navigate through multiple domains. The reason for this is actually rooted in how Cypress is designed. Instead of calling browser actions the same way as tools like Playwright and Selenium do, Cypress inserts the test script right inside the browser and automates actions from within. There are two iframes, one for the script and one for the application under test. Because of this design, browser security rules limit how these iframes interact and navigate. Laying grounds for solving these limitations were actually present in earlier Cypress releases and have finally landed in full with version 12 release. If you want to read more about this, you should check out Cypress’ official blog on this topic – it’s an excellent read.
There are still some specifics on how to navigate to a third party domain in Cypress, best shown by an example:
it('Google SSO login', () => {
cy.visit('/login') // primary app login page
cy.getDataCy('google-button')
.click() // clicking the button will redirect to another domain
cy.origin('https://accounts.google.com', () => {
cy.get('[type="email"]')
.type(Cypress.env('email')) // google email
cy.get('[type="button"]')
.click()
cy.get('[type="password"]')
.type(Cypress.env('password')) // google password
cy.get('[type="button"]')
.click()
})
cy.location('pathname')
.should('eq', '/success') // check that we have successfully
})
As you see, all the actions that belong to another domain are wrapped in the callback of cy.origin() command. This separates actions that happen on the third party domain.
The Cypress team actually developed this feature alongside another one that came out from beta, cy.session(). This command makes authenticating in your end-to-end tests much more effective. Instead of logging in before every test, you can log in just once, cache that login, and re-use it across all your specs. I recently wrote a walkthrough of this command on my blog and showed how you can use it instead of a classic page object.
This command is especially useful for the use case from the previous code example. Third-party login services usually have security measures in place that prevent bots or automated scripts from trying to login too often. If you attempt to login too many times, you might get hit with CAPTCHA or some other rate-limiting feature. This is definitely a risk when running tens or hundreds of tests.
it('Google SSO login', () => {
cy.visit('/login') // primary app login page
cy.getDataCy('google-button')
.click() // clicking the button will redirect to another domain
cy.session('google login', () => {
cy.origin('https://accounts.google.com', () => {
cy.get('[type="email"]')
.type(Cypress.env('email')) // google email
cy.get('[type="button"]')
.click()
cy.get('[type="password"]')
.type(Cypress.env('password')) // google password
cy.get('[type="button"]')
.click()
})
})
cy.location('pathname')
.should('eq', '/success') // check that we have successfully
})
When running a test, Cypress will make a decision when it reaches the cy.session() command:
- Is there a session called google login anywhere in the test suite?
- If not, run the commands inside the callback and cache the cookies, local storage, and other browser data.
- If yes, restore the cache assigned to a session called “google login.”
You can create multiple of these sessions and test your application using different accounts. This is useful if you want to test different account privileges or just see how the application behaves when seen by different accounts. Instead of going through the login sequence through UI or trying to log in programmatically, you can quickly restore the session and reuse it across all your tests.
This also means that you will reduce your login attempts to a minimum and prevent getting rate-limited on your third party login service.
Run all specs in GUI
Cypress GUI is a great companion for writing and debugging your tests. With the version 10 release, it has dropped support for the “Run all specs” button in the GUI. The community was not very happy about this change, so Cypress decided to bring it back.
The reason why it was removed in the first place is that it could bring some unexpected results. Simply put, this functionality would merge all your tests into one single file. This can get tricky especially if you use before(), beforeEach(), after() and afterEach() hooks in your tests. These would often get ordered and stacked in unexpected order. Take following example:
// file #1
describe('group 1', () => {
it('test A', () => {
// ...
})
})
it('test B', () => {
// ...
})
// file #2
before( () => {
// ...
})
it('test C', () => {
// ...
})
If this runs as a single file, the order of actions would go like this:
- before() hook
- test B
- test C
- test A
This is mainly caused by how Mocha framework executes blocks of code. If you properly wrap every test into describe() blocks, you would get much less surprises, but that’s not always what people do.
On the other hand, running all specs can be really useful when developing an application. I use this feature to get immediate feedback on changes I make in my code when I work on my cypress plugin for testing API. Whenever I make a change, all my tests re-run and I can see all the bugs that I’ve introduced. ?
Running all specs is now behind an experimental flag, so you need to set experimentalRunAllSpecs to true in your cypress.config.js configuration file.
Test isolation
It is always a good idea to keep your tests isolated. If your tests depend on one another, it may create a domino effect. First test will make all the subsequent tests fail as well. Things get even more hairy when you bring parallelisation into the equation.
You could say that Cypress is an opinionated testing framework, but my personal take on this is that this is a good opinion to have. The way Cypress enforces test isolation with this update is simple. In between every test, Cypress will navigate from your application to a blank page. So in addition to all the cleaning up Cypress did before (clearing cookies, local storage), it will now make sure to “restart” the tested application as well.
In practice the test execution would look something like this:
it('test A', () => {
cy.visit('https://staging.myapp.com')
// ...
// your test doing stuff
})
// navigates to about:blank
it('test B', () => {
cy.get('#myElement') // nope, will fail, we are at about:blank
})
This behavior is configurable, so if you need some time to adjust to this change, you can set testIsolation to false in your configuration.
Removing of deprecated commands and APIs
Some of the APIs and commands reached end of life with the latest Cypress release. For example, cy.route() and cy.server() have been replaced by the much more powerful cy.intercept() command that was introduced back in version 6.
The more impactful change was the deprecation of Cypress.Cookies.default() and Cypress.Cookies.preserveOnce() APIs that were used for handling the behavior of clearing up and preserving cookies. With the introduction of cy.session(), these APIs didn’t fit well into the system. The migration from these commands to cy.session() might not seem as straightforward, but it is quite simple when you look at it.
For example, instead of using Cypress.Cookies.preserveOnce() function to prevent deletion of certain cookies you can use cy.session() like this:
beforeEach(() => {
cy.session('importantCookies', () => {
cy.setCookie('authentication', 'top_secret');
})
});
it('test A', () => {
cy.visit('/');
});
it('test B', () => {
cy.visit('/');
});
Also, instead of using Cypress.Cookies.defaults() to set up default cookies for your tests, you can go to your cypress/support/e2e.js support file and set up a global beforeEach() hook that will do the same as shown in the previous example.
Besides these there were a couple of bug fixes and smaller tweaks which can all be viewed in Cypress changelog. Overall, I think that the v12 release of Cypress is one of the unsung heroes. Rewriting of query commands and availability of cy.session() and cy.origin() commands may not seem like a big deal on paper, but it will make the experience much smoother than it was before.
New command queries might require some rewriting in your tests. But I would advise you to upgrade as soon as possible, as this update will bring much more stability to your tests. I’d also advise to rethink your test suite and integrate cy.session() to your tests as it might not only handle your login actions more elegantly but shave off minutes of your test run.
If you want to learn more about Cypress, you can come visit my blog, subscribe to my YouTube channel, or connect with me on Twitter or LinkedIn.